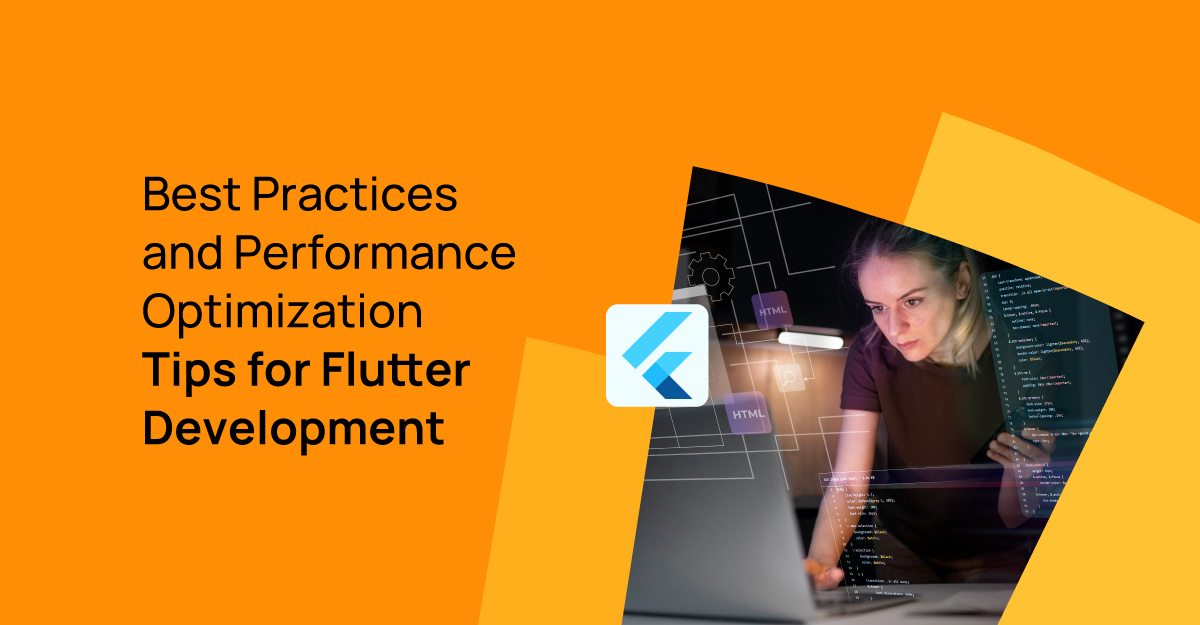
Flutter is an open-source framework for creating mobile applications that has become increasingly popular among developers and any Flutter development company, owing to its user-friendly and intuitive features. The fact that it can develop high-performing apps that function across different platforms is one of the primary factors contributing to its popularity. Like any other framework, there are still methods to further improve the performance of Flutter apps.
Why Flutter Best Practices are Essential for Developers
Following best practices in development is becoming more crucial as software development advances and technology progresses. By adhering to best practices, developers can enhance the code's quality, readability, maintainability, and robustness.
As everyone is on common ground with coding standards and conventions, adhering to best practices can improve developer collaboration and streamline productivity.
Without further ado, let's get started!
1. Leverage State Management
Efficient State Management State management plays a crucial role to boost the Flutter app performance. Choosing the right state management approach based on the complexity of your app is crucial to scaling the app appropriately. Multiple state management libraries are available, like BLoC, Provider, GetX, Riverpod, etc., of which BLoC is the most widely used and accepted.
Benefits of using State Management:
- Better Code Organization:
It helps in structuring the code in a more organized manner, making it easier to understand and maintain.
- Efficient Updates:
By managing the state separately from properties, you can achieve more efficient updates. Instead of rebuilding the entire app, Flutter only rebuilds the affected widgets when changes are made to the state. Efficient state management allows for reactive updates to the user interface. When the underlying data changes, the UI is automatically updated to reflect those changes without manual intervention.
- Improved App Performance:
Effective state management can lead to improved app performance.
- Testability:
State management techniques enhance the testability of the code. Well-managed state allows for easier unit testing of various components. By decoupling business logic and UI, you can test the logic independently, making it simpler to identify and fix bugs.
- Dynamic and Responsive Apps:
By managing state effectively, developers can create dynamic, responsive, and engaging apps.
- Scalability:
Good state management practices contribute to the scalability of your Flutter application. As the app grows in complexity, having a robust state management solution helps in maintaining a manageable and scalable codebase.
- Adaptability to Different Architectures:
Flutter supports a range of state management approaches, including Provider, Bloc, Redux, and more. This flexibility allows developers to choose the most suitable architecture based on the requirements of the project.
BLoC
This package is meant to help with the separation of business logic from user interface. Developers can concentrate on creating business logic by using this package, which encapsulates the reactive parts of the pattern.
Provider
It is a good idea to begin with this approach if you are new to Flutter. The Provider package uses limited code and is simple to understand. Also, it makes use of the same concepts as any other strategy.
GetX
GetX is a very lightweight and robust Flutter solution. It efficiently and practically integrates intelligent dependency injection, route management, and high-performance state management. It is concentrated on efficiency and low resource usage.
Riverpod
Similar to Provider, Riverpod is testable and compile-safe. Although it addresses several of Provider's main issues—supporting numerous providers of the same type, awaiting asynchronous providers, and adding providers from anywhere—it is still heavily influenced by Provider.
2. Adhere to Proper Naming Conventions
When naming variables and methods, use camelCase. Example : Instead of “first_name”, use “firstName”.
Give variables, methods, and classes appropriate names that accurately reflect their functions and purposes. Example : Using “UserModel” rather than “User”.
Classes, enums, typedefs name should be in UpperCamelCase.
Libraries, packages, directories, and source files names should be in snake_case(lowercase_with_underscores). Variables, constants, parameters, and named parameters should be in lowerCamelCase.
Strings : PREFER using interpolation to compose strings and values. Do not use + to build a string out of literals and other values. That does work in Dart, but it almost does not look clean & shorter.
Meaningful names for variables: Please avoid general names like value, test, mock instead of this please use email,userName.
Meaningful functions names :Use proper names that accurately describe what the function does. Avoid using common names like “doSomething” or “doCalculation.” Please use names like checkValidation, doWeeklyCalculations.
Meaningful constant names :Use names that accurately describe the value they represent. Avoid using generic names like “value” or “constant”.Please use constants like const double radius = 3 do not use const double constant = 3
Proper code formatting:
// Bad
void myDataFunction() {
// Complex and lengthy code here
}
// Good
void myDataFunction() {
doTask1();
doTask2(); doTask3();
}
Short functions for focused work :Functions that are focused on a single task and keep them short. This can help make your code more modular and easier to read instead of unwanted long code that is readable but it's too long. If functionality is too long then make 2 or 3 other functions & use it in the main function for neat & clean code.
// Bad
void myDataFunction() {
// Complex and lengthy code here
}
// Good
void myDataFunction() {
doTask1();
doTask2(); doTask3();
}
3. Widget Tree Optimization
We can avoid unnecessarily rebuilding of the widget tree by using const constructors for stateless widgets and implementing the shouldRebuild method for stateful widgets. We must thoughtfully make use of const keywords and use it as much as possible to optimize the performance and avoid rebuilding of any and every widget that does not require to be rebuilt.
4. Reusable Code Components
Reusability can be a key factor in optimizing the overall performance of the application. In Flutter app development, developing reusable code components is similar to building blocks that lay the foundation for scalable and maintainable applications.
Benefits of Reusable Components
- Code Reusability:
Components can be employed across the app, avoiding redundancy and development time significantly.
- Consistency in UI:
Consistent components across the app foster a unified look and feel, amplifying the user experience.
- Scalability Enabler:
Simplifies scaling the application as it grows, enabling seamless addition of new features without reworking existing components.
- Maintenance Simplicity:
Modular components streamline maintenance and updates. Changes to one component don't disrupt others if well encapsulated.
- Developer Efficiency Boost:
Once created and tested, reusable components expedite the development process for similar features or UI elements.
- Facilitating Debugging and Testing:
Smaller, reusable components are easier to debug and test due to their clear scope and purpose.
- Community Contribution and Sharing:
Well-abstracted components can be shared with the Flutter community, enriching the ecosystem of packages and libraries.
Example:
// CustomButton Component
import 'package:flutter/material.dart';
class CustomButton extends StatelessWidget {
final String text;
final VoidCallback onPressed;
final Color buttonColor;
final Color textColor;
const CustomButton({
required this.text,
required this.onPressed,
this.buttonColor = Colors.blue,
this.textColor = Colors.white,
});
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: onPressed,
style: ElevatedButton.styleFrom(
primary: buttonColor,
),
child: Text(
text,
style: TextStyle(color: textColor),
),
);
}
}
- The CustomButton exemplifies the principles of reusability, encapsulation, and flexibility by allowing customization of text, colors, and actions while maintaining a consistent design.
5. Lazy Loading and Pagination
When it comes to application development, efficiency and user experience are paramount. Flutter, known for its versatile toolkit, offers a powerful duo for handling copious data—Lazy Loading and Pagination.
Lazy loading is all About Chunking Data for Enhancing the Performance
Breaking down data into bite-sized chunks ensures that only the necessary portions are loaded, slashing initial load times and enhancing app responsiveness.
Streamlining with Throttling and Debouncing
Strategically controlling loading frequencies prevents excessive network calls, optimizing performance while minimizing unnecessary data consumption.
Pagination Controls for Smooth Navigation
Providing user-friendly controls enables effortless navigation between different data pages, enhancing accessibility and ease of use.
Efficient Error Handling
Managing loading errors gracefully with informative messages or retry options mitigates user frustration during network hiccups, ensuring a smoother experience.
Benefits: Streamlined Performance and Enhanced User Experience
Optimal Performance
Reduced initial load times and minimized memory usage elevate app responsiveness, offering swift and seamless user interactions.
Resource Optimization
Efficient data retrieval minimizes network consumption, conserving device resources and optimizing overall performance.
Improved User Experience
Facilitates smoother content navigation and quicker access to specific sections, enhancing overall user satisfaction.
Scalability
Effortlessly manages large datasets, ensuring consistent app performance and scalability for future growth.
Combining the prowess of lazy loading and pagination in Flutter applications elevates app efficiency, user experience, and scalability. Embracing these techniques aligns with the ethos of crafting high-performing, user-centric apps in today's competitive landscape.
6. Code Separation/Splitting
In Flutter development, keeping your codebase organized can make a world of difference. Here's a quick guide to tidy code separation in Flutter for a more manageable and cleaner app structure.
Multiple practices can be followed to separate / split the code based on:
-
Modularization:
Organize your code into distinct modules based on functionality, making it easier to navigate and maintain. Use directories or packages to keep different modules separated, aiding in better organization.
-
Widget Encapsulation:
Bundle UI components into separate widgets for better code isolation and reusability. Extract common UI elements into reusable widgets, reducing repetition and enhancing code clarity.
-
Separation of Concerns:
Divide your code based on specific responsibilities to improve readability and Maintenance. Keep business logic, UI, and data handling separate for clearer organization.
-
Dependency Management:
Efficiently manage dependencies, ensuring each module or component relies only on necessary dependencies. Use Flutter's dependency management system to keep dependencies clean and well structured.
-
Code Reusability:
Identify reusable code portions and create separate modules or functions for widespread use. Encourage code reuse to minimize duplication and improve code maintainability.
-
Documentation and Testing:
Document code effectively to help other developers understand and use it. Write unit tests for different modules or components to ensure functionality and stability
Benefits of Code Separation:
-
Easier Maintenance:
Organized code structure makes updates and modifications focused and Straightforward. Simplifies collaboration among team members, enabling parallel development on different modules.
-
Readability and Scalability:
Well-organized code improves readability and comprehension. Facilitates easy scalability by accommodating new features seamlessly.
-
Potential Performance Boost:
A clean code structure might contribute to better app performance by improving resource management.
7. Efficient Networking
Networking can be considered as the most important part of an Application and if not handled well, can lead to a tremendously bad User experience. To cater the same we can follow the below practices:
-
Utilize efficient network libraries
For networking we can use mostly two network libraries one is HTTP and another is Dio
How we can choose
- If you need a simple and easy-to-use package for basic HTTP requests,Http is a good choice.
- But if you need a more powerful and feature-rich package, Then we should to use Dio
Always there is some advantages and some disadvantages
HTTP:
- Advantages
- HTTP is lightweight and easy to use, making it ideal for small projects.
- Provided by the Dart developers
- Disadvantages
- Only provide basic functionality
- Error handling is complex
Dio:
1. Advantages
- Dio is fast and efficient due to its support for connection pooling and request cancellation.
- Dio supports multiple concurrent requests, making it ideal for handling multiple API calls.
- In addition to performing basic network stuff, dio also provides some extra functionality like interceptors, log, cache, etc;
-
-
Implement caching
a. Caching is a crucial process for storing API responses on a user's device to reduce network requests and improve performance in Flutter apps.
b. Dio Cache Interceptor: An interceptor for Dio that allows efficient API response caching.
i. Example :
var customDio = Dio()..interceptors.add(DioCacheInterceptor(options: customCacheOptions));
-
Data compression
a. Implementing data compression is an effective strategy to reduce the size of data transmitted over the network, resulting in improved network performance in Flutter applications.
b. Add the flutter_zlib package to your pubspec.yaml file: c. Example:
void sendCompressed() async { var gzip = GZipCodec(); String jsonToSend = '{"field1":"Some JSON to send to the se var compressedBody = gzip.encode(jsonToSend.codeUnits); Map<string, string=""> headers = { "Content-Encoding": "gzip", " Content-Type": "application/json; charset=UTF-8", }; var response = await http.post( "<a href="<a href=" https:="" example.com="" api="" v1""=""></a><a href=" https://example.com/api/v1 " <="" a="">"> https://example.com/api/v1 "</a></a> ,"=""><a href="<a href=" https:="" example.com="" api="" v1""=""> https://example.com/api/v1 "</a> ,<="" a="">"><a href=" https://example.com/api/v1 " ,<="" a=""></a>"><a href=" https://example.com/api/v1 " ,<="" a=""></a>"><a href=" https://example.com/api/v1 " ,<="" a=""></a>"> https://example.com/api/v1 ",</a></a> headers, compressedBody ); } </string,>
d. Proper concurrency management
a. a. Combining requests efficiently can enhance performance, reduce network overhead, and improve user experience.
i. Sequential Combination
Example:
Future<List<dynamic>> combineRequestsSequentially() async { List<dynamic> results = []; var result1 = await makeRequest1(); results.add(result1); var result2 = await makeRequest2(); results.add(result2); return results; }
ii. Sequential Combination
Future<List<dynamic>> combineRequestsInParallel() async { List<Future<dynamic>> futures = []; futures.add(makeRequest1()); futures.add(makeRequest2()); futures.add(makeRequest3()); List<dynamic> results = await Future.wait(futures); return results; }
e.Request optimization
Request optimization is a critical aspect of improving network performance in Flutter applications. By optimizing requests, you can reduce the amount of data transferred over the network, minimize the number of requests made, and enhance overall efficiency. You can use below some strategies
1. Result pagination
2. Image compression
-
Implement caching
8. Code Commenting
Dart supports three kinds of comments:
// Inline comments
/*
Blocks of comments. It's not convention to use block comments in
*/
/// Documentation
///
/// This is what you should use to document your classes.
So some explanations about what a line of code does within a method, we would use the double slash // , while those comments that explain what a method does, or what a property is for or what a class does (these three groups should be in a generated documentation) we would use the triple slash /// .
9. Screen Size Optimization
There are two basic approaches to creating Flutter apps with responsive design:
- Use the LayoutBuilder class : The LayoutBuilder widget is a powerful tool in Flutter optimization that can help us create responsive designs. It provides a builder property that passes the current BuildContext and BoxConstraints.
LayoutBuilder( builder: (BuildContext ctx, BoxConstraints constraint return Container( color: Colors.green, /// Aligning contents of this Container /// to center alignment: Alignment.center,<p> /// Using parent's constraints /// to calculate child's height and width height: constraints.maxHeight * 0.5, width: constraints.maxWidth * 0.5, child: Text( 'LayoutBuilder Widget', style: TextStyle( fontSize: 18, fontWeight: FontWeight.bold, color: Colors.white, ), ), ); },</p>
- Use the MediaQuery.of() method:
Apart from the above two, we can also keep in mind the below implementation while developing for Flutter Apps.
Orientation Builder:
The OrientationBuilder widget is another useful tool for creating responsive layouts in Flutter. It provides a builder property that passes the current BuildContext and Orientation. The Orientation object tells us whether the current device orientation is portrait or landscape.
OrientationBuilder( builder: (BuildContext context, Orientation orientation) { if (orientation == Orientation.landscape) { return _buildWideContainers(); } else { return _buildNormalContainers(); } }, ),
Aspect Ratio:
The AspectRatio widget in Flutter is used to adjust the aspect ratio of a widget. The aspect ratio is defined as the ratio between the width and the height of a box
Use Built-In Responsive Widget:
- Flexible: Flutter's Flexible widget effortlessly adjusts to available space, making your app layout adapt dynamically for a seamless and responsive user experience.
- Expanded: Improve Flutter app's layout with the Expanded widget, efficiently utilizing available space to ensure a visually balanced and appealing design
Conclusion
These best practices for Flutter optimization and app development can help you develop cross-platform apps even more effectively. Flutter is one of the latest solutions for app development.
The process of creating apps can be made simpler by leveraging these Flutter best practices.
Consider hiring Flutter developers for efficient Flutter app development services if you're facing issues with development.